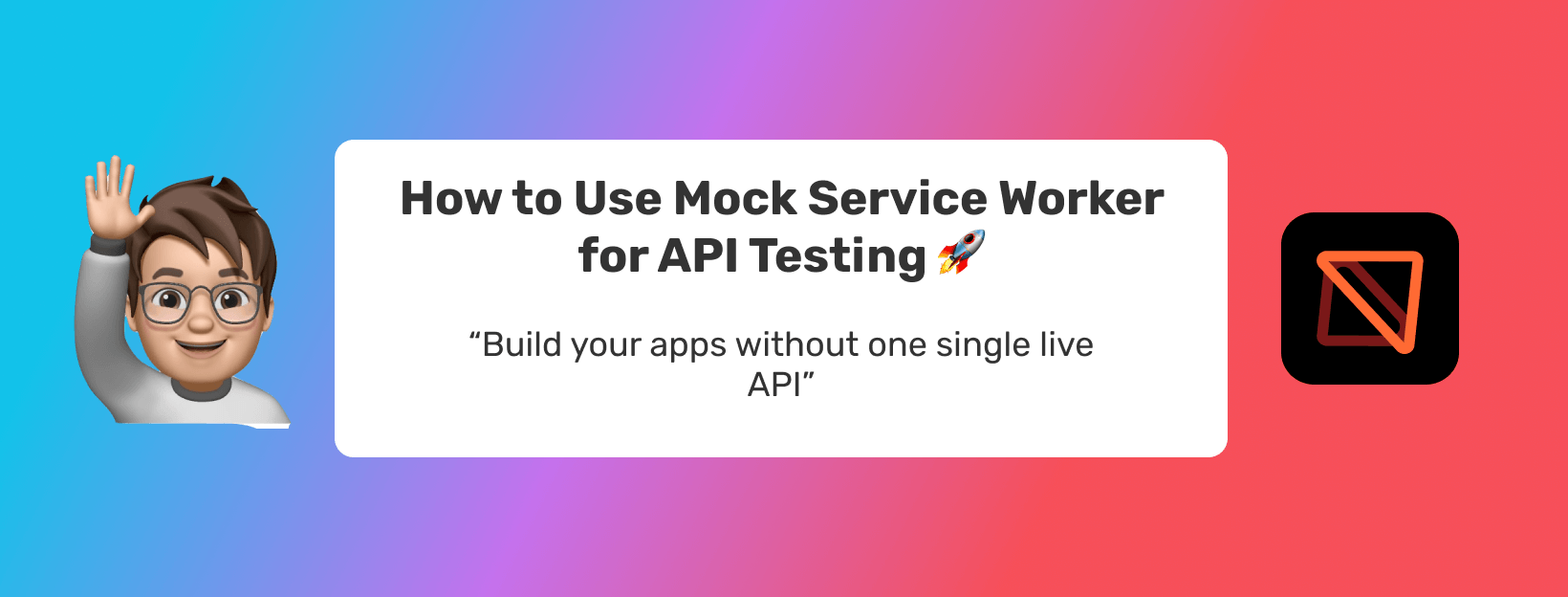
How to Implement Dark Mode in your application in 3 easy steps
In this article, we're going to see how we can implement dark mode in our website. Before we get into the meat and potatoes of the article, lets try and understand why we might want to implement Dark Mode.
Date Posted : 13 November 21
Time to read: 6 mins
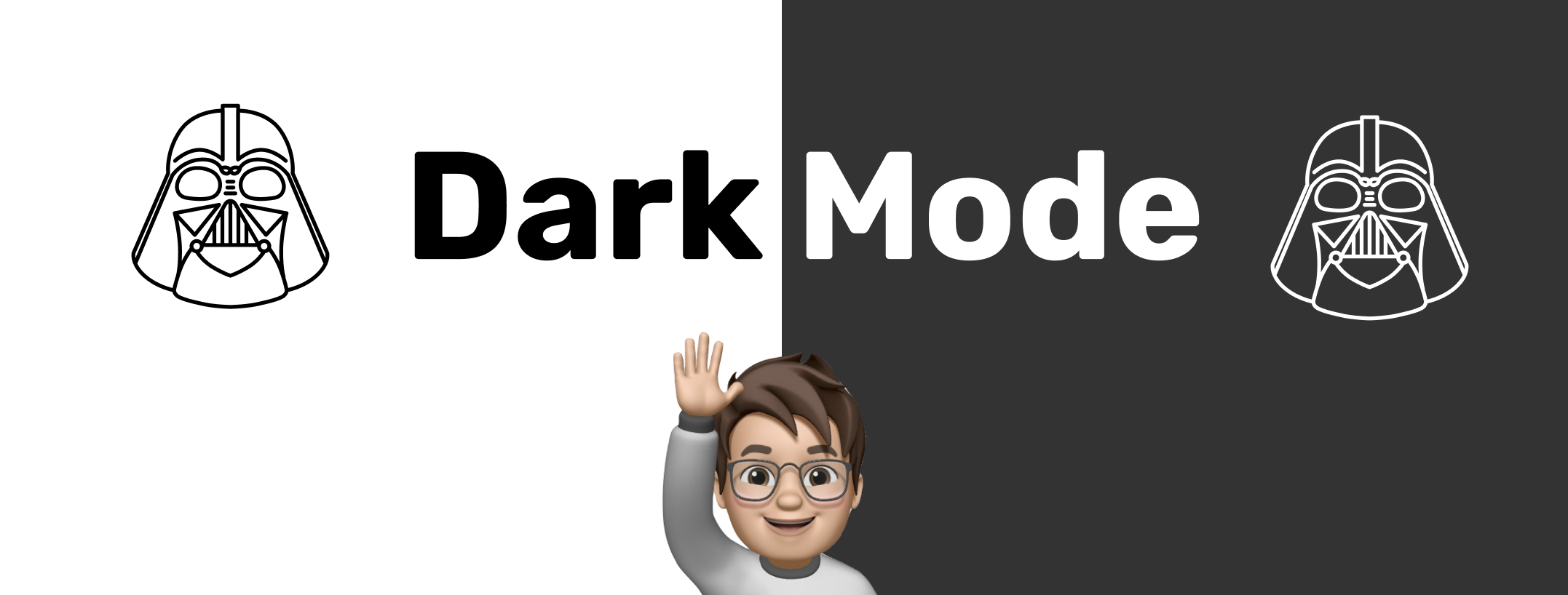
In this article, we’re going to see how we can implement dark mode on our website. Before we get into the meat and potatoes of the article, let’s try and understand why we might want to implement Dark Mode.
Why Dark Mode anyway?
Reduces Strain on the Eyes
- With the ever-growing usage of Digital Technology and more and more exposure to screens, one very frequent problem among users is the strain that’s caused due to the exposure to light from these devices. To cite this, according to American Optometric Association, around 58% of adults have experienced “Digital Eye Strain” related to using more devices. Some users claim to find Dark Mode more comfortable for their eyes.
Reduces Blue Light Exposure at night
- Blue Wavelengths- which are beneficial during daylight hours as they help with attention and mood, seem to be disruptive at night. And with more and more device screens, we’re exposed to blue light more than ever.
- Blue Light has been shown to disrupt sleep patterns by decreasing the secretion of melatonin.
More Users prefer it
- According to research by Android Authority and polar, around 92% and 95% of participants respectively declared their preference for dark mode.
- Also, more and apps are adding support for Dark Mode like Facebook, LinkedIn, Twitter, Reddit to name a few.
Doesn’t Eat your Battery
- Dark Mode improves battery life for devices with OLED screens. The reason for this is when it comes to OLED screens as the screen lights up colored pixels only while leaving the black pixels turned off. This means a dramatic increase in battery life and greater longevity for their battery in the long term
{{< image title=“Dark Mode” alt=“Dark Mode” src=”https://media.giphy.com/media/MtGAE4ZabBs4g/giphy.gif” >}}
Implementation Overview
Before we begin, I’m just going to lay down the steps we’re going to follow to implement the Dark Mode
- Defining CSS variables for each mode
- Creating Elements that use colors from CSS Variables
- Hooking everything up with Javascript
Step 1: Defining colors for each theme
- One of the most important things to remember while building an application be it with the Dark Mode or without it is to follow a style guide that dictates what colors will be used throughout your application. This forms the basis for the theme your application is going to use.
- Let’s define the colors for each of the modes in our styles.css file
// For Normal Theme
:root {
--primarytext: #333333;
--secondarytext: #797779;
--bgcolor: white;
--greylight: #f6f6f6;
}
// For Dark Theme
[data-theme="dark"] {
--primarytext: #ffffff;
--secondarytext: #b0b3b8;
--bgcolor: #18191a;
--greylight: #232526;
}
- What we’re essentially doing here is defining CSS Variables and we’re saying that we want to use the first set of variables for when the user is in Light Mode (Default) and to use the second set of variables for when the user switches to Dark Mode
- As you can see, it’s very important to keep one thing into consideration here. For each color defined under the ‘root’ variables, we have an equivalent variable defined in the dark mode set of variables
Step 2: Creating Elements which use colors from the defined CSS Variables
Now, that we have our themes defined. Let’s go ahead and create a few HTML elements. For the sake of this article, I’m just going to use the CSS variables on the following elements
- Header
- Paragraph
- Card Element (Custom element that we’re going to create)
h1{
color: var(--primarytext)
}
p{
color: var(--primarytext)
}
.card{
padding:20px;
width : 200px;
height: 200px;
backgroundColor: var(--greylight)
}
As you can see from the code above, we’re using the CSS variables to add color to the header, paragraph elements, and the card class. By default, the values for the variables will be picked up from the variables defined under the root styles, but we’ll use javascript in the next step to change it to point to the variables defined under the dark theme. Let’s see how we can achieve that.
Step 3: Hooking everything up with Javascript
In this step, let’s use javascript to dynamically toggle the mode as and when a certain event is detected (example, button click). We’ll also be persisting the users’ selected mode in LocalStorage so that the next time the user opens the page, the theme is the one that the user selected before. Also, for the sake of this article, we’ll just be implementing the dark mode on the client-side. We can take this one step further by persisting the user’s selected mode in the database which is the preferred approach.
Enough talking, lets see the code now
const toggleButton = document.getElementById("toggle");
function changeTheme(mode) {
document.documentElement.setAttribute("data-theme", mode);
}
function changeMode() {
let currentMode = localStorage.getItem("mode");
if (currentMode === "dark") {
changeTheme("light");
localStorage.setItem("mode", "light");
}
if (currentMode === "light") {
changeTheme("dark");
localStorage.setItem("mode", "dark");
}
}
toggleButton.addEventListener("click", changeMode);
As we can see from the code above, we trigger the change mode method every time the button is clicked on. This checks the localstorage to get the current mode, the mode is then reversed. Also, note that we’re using document.documentElement.setAttribute()
which changes the theme to the specified value.
To see a working example of the project you can head over to the following Codesandbox link.
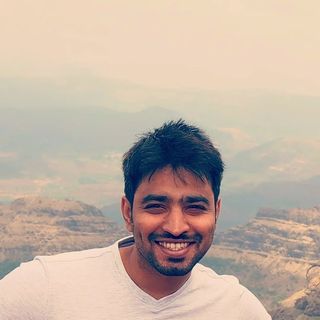
Shivaraj Bakale
Diving deep from frontend waves to the backend caves 🌊🤠
React
Redux
Redux-saga
HTML