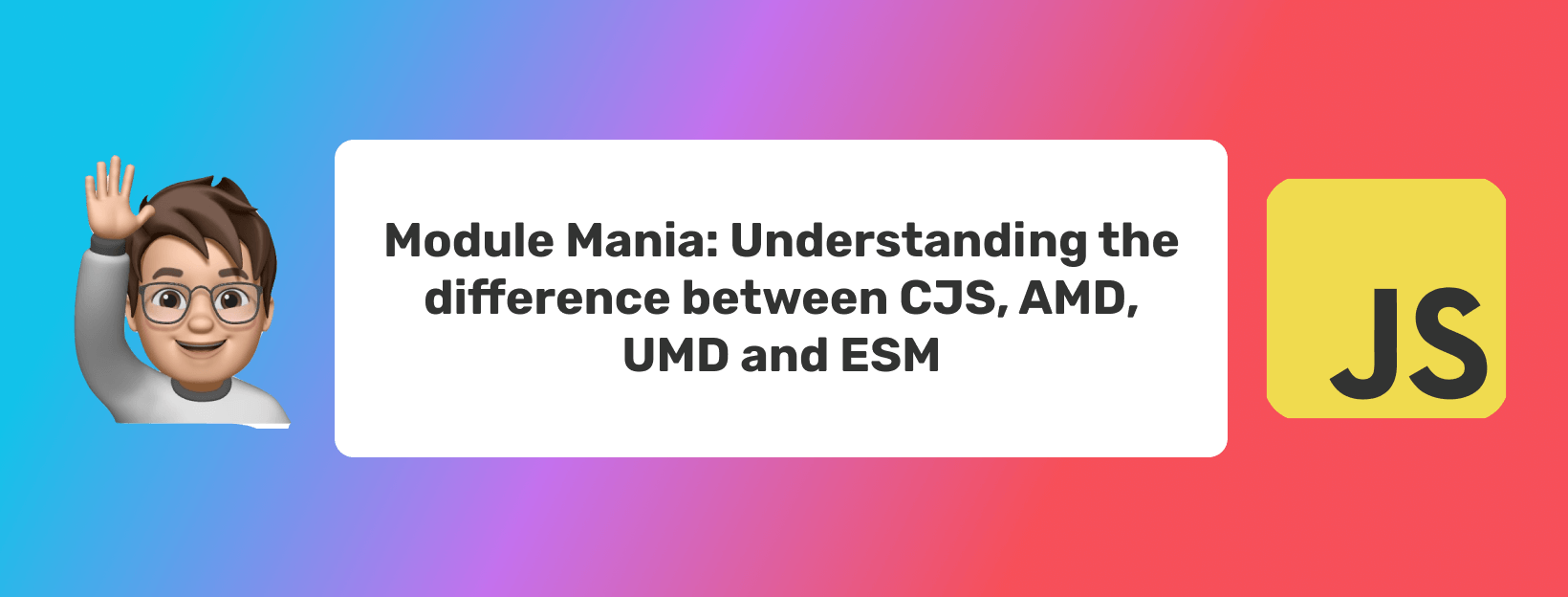
How to Use Mock Service Worker for API Testing 🚀
When it comes to testing APIs, one of the key challenges is ensuring that your application behaves correctly under all conditions. Simulating different scenarios, such as server errors or slow response times, can be difficult. That's where Mock Service Worker (MSW) comes in. MSW is a powerful tool that allows you to mock HTTP requests and responses in your tests, making it easy to test your application's behavior under different circumstances. In this article, I'll show you how to use MSW to test your APIs, step by step.
Date Posted : 03 September 23
Time to read: 8 mins
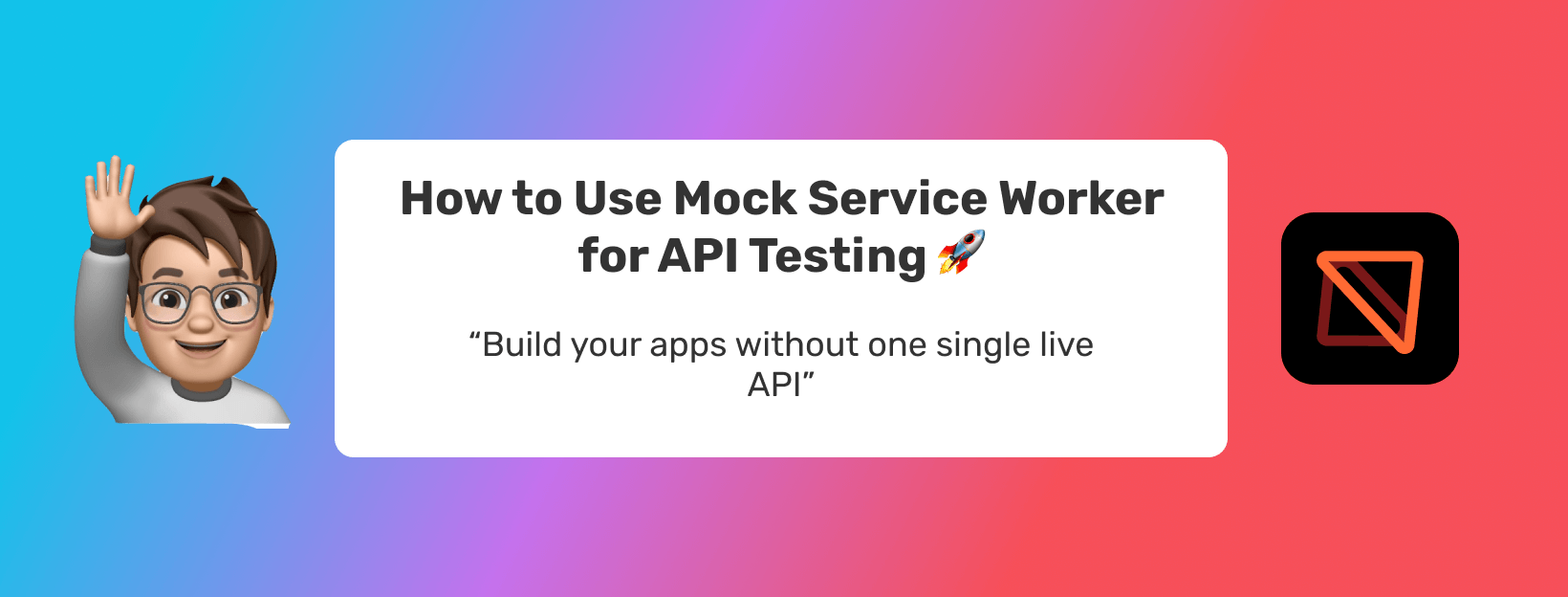
Introduction
When it comes to testing APIs, one of the key challenges is ensuring that your application behaves correctly under all conditions. Simulating different scenarios, such as server errors or slow response times, can be difficult. That’s where Mock Service Worker (MSW) comes in. MSW is a powerful tool that allows you to mock HTTP requests and responses in your apps and tests, making it easy to test your application’s behavior under different circumstances. In this article, I’ll show you how to use MSW to test your APIs, step by step.
What is Mock Service Worker?
Mock Service Worker is a library that allows you to mock HTTP requests and responses in your tests. It works by intercepting requests made by your application and returning mock responses that you define. By simulating different scenarios, such as server errors or slow response times, you can ensure that your application behaves correctly under all conditions. Also, a common scenario is where the API’s front end backend team are not ready yet and one needs a way in which one can mock the responses, MSW to the rescue.
Sample use cases for using MSW
- Testing APIs in your applications.
- Simulating different scenarios (such as server errors or slow response times) to ensure that your application behaves correctly under all conditions.
- Defining mock responses for your API requests.
Setting Up MSW
To get started with MSW, you’ll need to install it using npm or Yarn. Here’s how:
npm install msw --save-dev
or
yarn add msw --dev
Once you’ve installed MSW, you can create a new server instance in your test file. This instance sets up a new server and starts it listening for requests. It also resets the server’s request handlers after each test and closes the server when all tests have completed.
import { setupServer } from 'msw/node'
const server = setupServer()
beforeAll(() => server.listen())
afterEach(() => server.resetHandlers())
afterAll(() => server.close())
Or one can simply go through the following link to use the CLI to set up MSW without manual intervention.
Writing Request Handlers
The next step is to define request handlers for your server. These handlers will intercept requests made by your application and return mock responses. You can define handlers for all types of HTTP requests, including GET, POST, PUT, and DELETE.
import { rest } from 'msw'
const handlers = [
rest.get('/user', (req, res, ctx) => {
return res(
ctx.json({
username: 'john.doe',
}),
)
}),
]
This code defines a request handler for a GET request to the /user
endpoint. When a request is made to this endpoint, the handler returns a JSON response containing a username.
Using MSW in a React Component
You can use MSW in your React components to ensure that your application behaves correctly under all conditions, including different scenarios such as server errors or slow response times. Here’s an example of how to use MSW in a React component:
import React, { useState, useEffect } from 'react'
import { rest } from 'msw'
function User() {
const [username, setUsername] = useState('')
useEffect(() => {
const fetchUsername = async () => {
const response = await fetch('/user')
const data = await response.json()
setUsername(data.username)
}
fetchUsername()
}, [])
return <div>{username}</div>
}
export default User
This code defines a User
component that fetches the username data from the server using MSW. When the component mounts, it makes a GET request to the /user
endpoint, which is intercepted by MSW and returns the mock response that you defined earlier. The component then sets the retrieved username to its state and renders it.
Make sure to set up your MSW server and define your request handlers before using them in your React components. You can use the setupServer
function from MSW in your test file to start the server before your tests, then use it to intercept requests in your component.
Using MSW in Your Tests
Now that you have set up your server and defined request handlers, you can start using MSW in your tests. Here’s an example:
test('fetches user data', async () => {
render(<App />)
const response = await fetch('/user')
const data = await response.json()
expect(data.username).toEqual('john.doe')
})
This code tests that your application can fetch user data from the server. When the fetch request is made, MSW intercepts it and returns the mock response that you defined earlier.
Additional Configuration Options
In addition to the basic configuration options I’ve covered so far, MSW offers a number of other configuration options that you can use to fine-tune your testing environment. Here are a few examples:
Setting status codes
The code below sets the status code of the response to 404 (Not Found) and returns a JSON response containing an error message. This is an example of how to set status codes using MSW in your API tests.
const handlers = [
rest.get('/user', (req, res, ctx) => {
return res(
ctx.status(404),
ctx.json({
errorMessage: 'User not found',
}),
)
}),
]
This code sets the status code of the response to 404 (Not Found) and returns a JSON response containing an error message.
Delaying responses
This code is delaying the response by 2000ms (2 seconds) before returning a JSON response containing a username. It is an example of how to use MSW to simulate slow response times in API testing.
const handlers = [
rest.get('/user', (req, res, ctx) => {
return res(
ctx.delay(2000),
ctx.json({
username: 'john.doe',
}),
)
}),
]
Conclusion
Mock Service Worker is a powerful tool for testing APIs in your applications. By simulating different scenarios, you can ensure that your application behaves correctly under all conditions. With MSW, you can easily define mock responses for your API requests, making it easy to test your application’s behavior. If you’re looking to improve the quality of your API testing, give MSW a try! Caio!! 👋
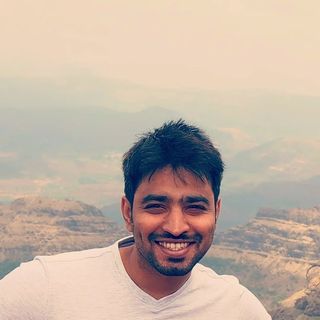
Shivaraj Bakale
Diving deep from frontend waves to the backend caves 🌊ðŸ¤
React
Redux
Redux-saga
HTML